Laravel Microsoft Graph: How to integrate API
- Nobody
- Jan 15
- 3 min read
Updated: Jan 24
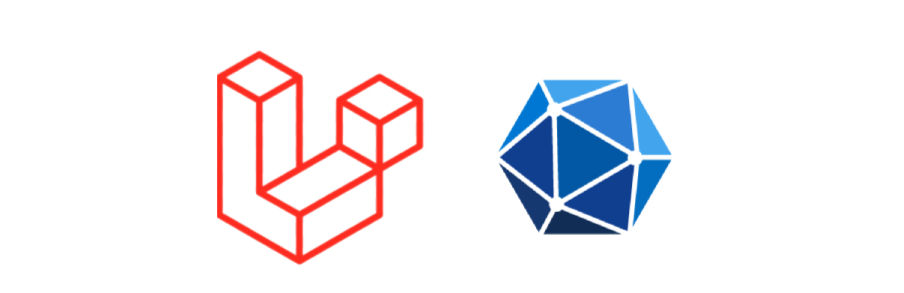
In this tutorial, I will demonstrate how to integrate the Microsoft Graph API with a Laravel application.
To interact with the Microsoft Graph API, I will implement the OAuth2 authorization code flow.
This tutorial consists of two parts:
Setting up user authentication with a Microsoft Azure application.
Calling Microsoft Graph API endpoints on behalf of an authorized user.
Before we start
To follow this tutorial, you will need:
A Microsoft Azure application to access the Microsoft Graph API.
A new Laravel project (official docs).
Setting Up Authentication For Microsoft Graph
Getting started
We’ll begin with a homepage to display the current user’s status and include links for authenticating the user and integrating the Microsoft Graph API.
Note: To keep this tutorial simple and focused on the Microsoft Graph API, I will not implement full Laravel user authentication.
Create a new homepage template home.blade.php with the following content:
In this view, user navigation links will be displayed based on the authentication status.
Update the root route in web.php to render the previously created view:
Authorizing the User Using OAuth2 Code Flow
To handle Microsoft Azure authorization, define a new service that encapsulates the necessary logic. Create an AuthorizationService class with the following code:
This service includes two main methods:
getAuthorizeUri: Builds the authorization URL.
exchangeTokens: Exchanges the authorization code response for access tokens.
Configuring Microsoft Azure application OAuth2 client
To begin, create a new OAuth2 client for your application. Copy the following properties from your Azure application secrets.
Next, navigate to the Microsoft Azure application dashboard and add the redirect_uri from your configuration to the whitelisted redirect URIs. This setup ensures secure communication between your application and Microsoft Azure while allowing it to retrieve user authorization tokens.
At this stage, we’ve created the authentication service and configured our application’s OAuth2 client. The next step is to define endpoints to initiate the authorization flow from the UI.
Creating Authorization Endpoints
Redirecting Users for Authorization
To redirect the user to the Microsoft Azure authorization page for actual authorization, add a new endpoint to your web.php file:
Handling Authorization Callback
After the user authenticates, Microsoft Azure sends back an authorization code response. This code must be exchanged for access and refresh tokens. Add the following code for the authorization callback endpoint:
This endpoint:
Exchanges the authorization code for tokens.
Stores the user’s authentication context in the session.
Redirects the user to the homepage.
Logging Out and Clearing Session
To allow removal of the current authorization context during testing, add an endpoint to clear the session:
Testing User Authentication
Start the application server.
Click the "Authorize" link on the homepage. You should be redirected to the Microsoft OAuth authorization page.
After authorizing the application, you’ll see "My Graph API Profile" and "Logout" links on the homepage.
Making Laravel Microsoft Graph API requests
Creating GraphServiceClient from Authorization Tokens
To interact with the Microsoft Graph API, create a factory class that instantiates GraphServiceClient using the tokens stored in the user's session. This factory uses the microsoft-graph-php library to initialize the client.
Here’s the code for the AppMicrosoftGraphClientFactory class:
Fetching and Displaying User Profile
Create a new my-profile.blade.php view to display the user profile:
Define a profile endpoint in web.php that uses AppMicrosoftGraphClientFactory to create the Graph API client and fetch user data:
Testing the Complete Integration
To test the full integration:
Start the application server.
Navigate to the homepage and click the "Authorize" link to initiate Microsoft OAuth authorization.
After completing authorization, a "My Graph API Profile" link should appear.
Click the profile link to view the authorized user details retrieved using the Graph API.
Now you can use the Microsoft Graph API client to fetch any resource of your choice. Ensure the scopes parameter includes the necessary permissions for accessing those resources.
Wrap-Up
In this tutorial, we successfully:
Integrated the Microsoft Graph API into a Laravel application.
Configured user authentication using OAuth2.
Called Microsoft Graph API endpoints to fetch user data.
In future tutorials, I’ll explore advanced topics, such as creating Microsoft Graph API change notification subscriptions.
What's Next
In the next post, I will create a Microsoft Graph API change notifications subscription to listen for real-time updates.
API Connects is a global IT services firm in New Zealand brand excelling in Technology Architecture, Consulting, Software development & DevOps. Consult today! Visit: https://apiconnects.co.nz/devops-infrastructure-management/