Send Parallel API Requests with Ruby on Rails
- Nobody
- Jan 16
- 1 min read
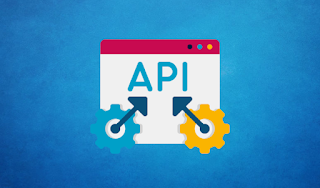
Often, in our business logic, we need to fetch data from multiple API resources before we can proceed with further processing. Executing each request sequentially is highly inefficient in terms of resource usage and time.
In this tutorial, I will describe how to use the Typhoeus library to execute a batch of requests in parallel.
Installing gem
Add this line to your Gemfile:
Creating Test API Service
In this tutorial, I will send parallel 5 requests to the jsonplaceholder fake API server.
Let's create an ApiService class with a get_request method that returns a new Typhoeus::Request object for a GET method and a dynamic URL. This request object is lazy and won't be executed until it is run explicitly or added to the scheduler.
Sending parallel requests
This method creates a Typhoeus::Hydra scheduler object, iterates over each URL, builds a GET request from it, and enqueues the requests into the scheduler.
After queuing all requests, we need to run the scheduler using the run method. This method executes all requests synchronously, and you can access the response data using the response method on the request object. Alternatively, you can specify an on_complete callback when creating the request.
Updated ApiService class
Now you can execute this logic using:
Essentially, that's all you need to execute API requests in parallel.
Typhoeus supports a wide range of configuration options that allow you to customize the concurrency level, error-handling logic, retry mechanisms, and more.
Comments